Dictionaries in Python are incredibly versatile data structures that offer various functionalities. Here are some interesting facts about dictionaries in Python:
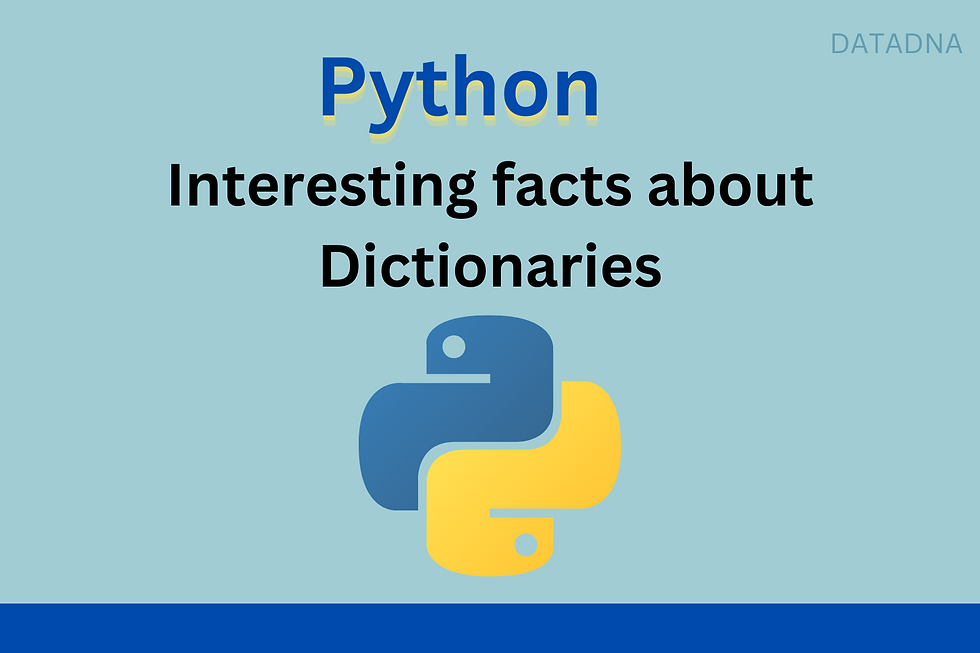
Key-Value Pairs: Dictionaries are composed of key-value pairs, where each key is unique within the dictionary and associated with a corresponding value. This allows for efficient lookup and retrieval of values based on their keys.
Mutable: Dictionaries are mutable, meaning you can modify their contents after creation. You can add new key-value pairs, remove existing ones, or update the values associated with existing keys.
Unordered: In Python versions before 3.7, dictionaries were unordered collections, meaning the order of elements was not guaranteed. However, starting from Python 3.7, dictionaries maintain the order of insertion, effectively preserving the order in which items are added.
Fast Lookup: Dictionaries offer fast lookup times for retrieving values based on keys. This is because they use a hash table implementation internally, which allows for constant-time average-case lookup complexity.
Flexible Key Types: Keys in dictionaries can be of various data types, including integers, strings, tuples (if they contain only immutable elements), and even custom objects if they implement the `__hash__()` and `__eq__()` methods correctly.
Immutable Keys: Keys in dictionaries must be immutable objects, meaning they cannot be modified after creation. This restriction ensures that the integrity of the dictionary remains intact, as mutable keys could lead to unexpected behavior.
Dictionary Comprehensions: Similar to list comprehensions, Python supports dictionary comprehensions, allowing you to create dictionaries using concise and readable syntax.
View Objects: Python dictionaries provide view objects (`dict_keys`, `dict_values`, and `dict_items`) that allow you to access the keys, values, and key-value pairs, respectively. These view objects provide dynamic views into the dictionary's contents and reflect changes made to the dictionary.
Memory Efficiency: Dictionaries are memory-efficient data structures, especially when compared to lists. They consume memory proportional to the number of elements they contain rather than allocating a fixed-size block of memory.
Popularity: Dictionaries are widely used in Python programming due to their versatility and efficiency. They are used in various applications, ranging from storing configurations and caching data to representing complex data structures and mapping relationships between entities.
Comments